This guide to walk through you to create API service using node.js from scratch. Node.js is a developer choice for creating APIs for mobile or web applications.
I’d recommend that you try each step and don’t move to the next step until the step is completed.
Case Study
Create an API for CRUD operations to manage TODO List stored in the MongoDB database.
Setup Project
We’ll start from an empty folder on your computer and will install all the required libraries and then move to the coding part.
Create a folder ‘todolist’ and go to the folder.
mkdir todolist
cd todolist
Initialize the project using ‘npm init’ and enter ‘todoapi’ as the name of the package and leave all other fields default.
npm init
and enter ‘yes’ to finish the initialization process. A new ‘package.json’ file is created to manage all package dependencies.
Now install node.js packages required for the project.
npm install mongoose --save-dev
npm install express --save-dev
npm install body-parser --save-dev
- mongoose – To access the MongoDB database.
- express – To handle the HTTP requests.
- body-parse – To handle the Posts data.
Setup Server
Create a server.js file and put the below code.
var express = require('express')
var app = express()
var port = 4000
app.listen(port)
Setup Database
mLab is a Database-as-a-Service for MongoDB so instead of installing MongoDB locally, we can use this and create a sample todo database.
- First login into mLab and click on ‘Create New’.
- Choose the Sandbox Plan and click on ‘Continue’
- Choose the ‘US East (Virginia)’ region and click on ‘Continue’
- Enter database name ‘mytodos’ and click on ‘Continue’
- Now click on ‘Submit Order’ to finish the setup.
- You’ll be redirected to the database dashboard.
- A database user is required to connect to this database so create a user first. To create one now, go the ‘Users’ and click the ‘Add database user’.
So we have created a sample MongoDB database to use in our application.
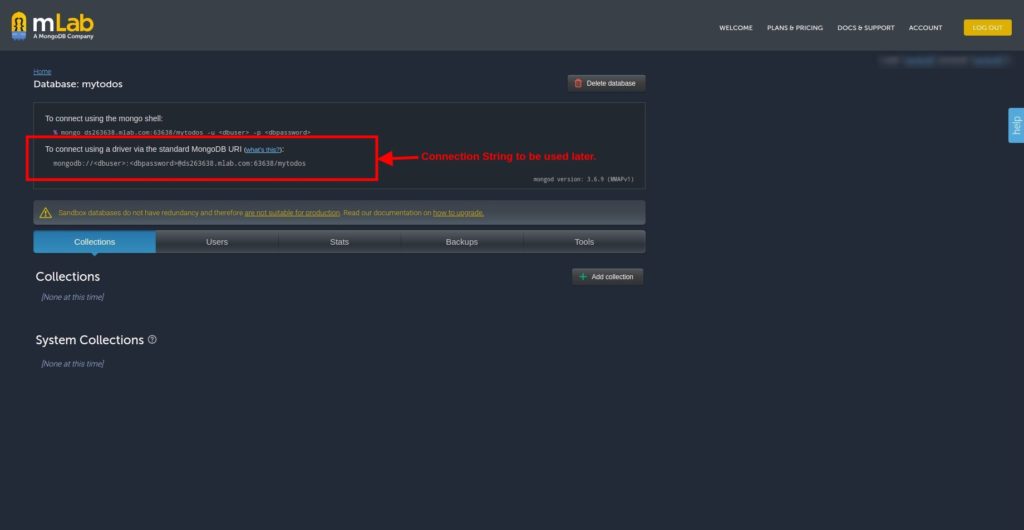
Setup Database Schema
In NoSQL, documents based database, we define the document schema to store the information. Let’s create it now.
Create a file ‘todoschema.js’ and put the below code into it.
var mongoose = require('mongoose')
var Schema = mongoose.Schema
var todoSchema = new Schema({
creator: String,
task: String,
isCompleted: Boolean
});
var Todos = mongoose.model('Todos',todoSchema)
module.exports = Todos
Here we created 3 fields in our document.
- creator – To store the user’s information.
- task – Describe the task to be done.
- isCompleted – has the task been completed or not?
Database Connection
It’s pretty easy to access the database we created on mLab. We just need to pass the connection string to the mongoose.connect() method. Open ‘server.js’ and put the below code.
username = 'admin'
password = 'a123456'
connectionString ="mongodb://"+username+":"+password+"@ds263638.mlab.com:63638/mytodos";
mongoose.connect(connectionString)
Use BodyParser
Use the body-parser library to handle the get and post data and convert them into a JSON object.
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({extended:true}))
Create Endpoint
Each APIs service provides an endpoint URL to send data or retrieve data via HTTP requests. It’s easy to create an endpoint URL using the Express library we installed.
// Test end Point
app.get('/api',function(req,res){
res.send('API is ready to receive HTTP requests.')
})
For testing, run the “node server.js” and hit the 127.0.0.1:4000/api in the browser and you should see “API is ready to receive HTTP requests” message on the browser window.

CRUD Methods
Now let’s write the main API part and write Create Todo, Retrieve Todos, Update Todo, and Delete Todo methods.
Insert Record
Use the below code to insert a new todo record into MongoDB.
// Insert Record
Todos = require('./todoschema.js')
app.get('/api/todo',function(req,res){
var testData = [
{
creator: 'sandeep',
task: 'Write function to insert record.',
isCompleted : false
}
]
Todos.create(testData,function(err,results){
res.send(results)
})
})
Though, usually, a post request is sent to the server to save the data so you should use the post method.
app.post('/api/todo',function(req,res){
var testData = [
{
creator: req.body.user,
task: req.body.task,
isCompleted : req.body.isCompleted
}
]
Todos.create(testData,function(err,results){
res.send(results)
})
})
Update Record
This will be a post request and ID should be passed to update the record.
app.post('/api/todo',function(req,res){
if(req.body.id) {
Todos.findByIdAndUpdate(req.body.id,{
task: req.body.task,
isCompleted:req.body.isCompleted
}, function(err,todo) {
if(err) {
throw err;
}
res.send('Record Updated.')
})
}
})
Delete Record
This will be a DELETE request and ID is mandatory.
app.delete('/api/todo',function(req,res){
if(req.body.id) {
Todos.findByIdAndRemove(req.body.id,function(err) {
if(err) {
throw err;
}
res.send('Record Deleted.')
})
}
})
Get Record
Use the findByID method to get a todo using the ID.
app.get('/api/todo/:id',function(req,res){
Todos.findById({_id: req.params.id},function(err,todo){
if( err ) {
throw err;
}
res.send(todo);
})
});
You can create simple to advanced APIs services by getting ideas from the above guide. Here is a complete list of functions you can use to deal with MongoDB.