In this guide, we’re going to create our own web server using Node.js which can respond back to an HTTP request.
Node.js is popular because you can write both server-side and client-side code using a single language Javascript. That’s why Javascript developers are in high demand.
Let’s start! Create a webserver.js file and put all the below codes into that.
Load HTTP Module
The first step is to load the HTTP module to create a web server.
var http = require('http');
Request Listener
Define a request listener who will give a response back to an HTTP request and will tell the browser the type of the contents as well.
var listener = function(req,res){
res.writeHead(200,{ 'Content-Type' : 'text/plain' })
res.end('Hello World\n');
}
Here we define the mime-type of contents being sent back to the HTTP request and sending a Hello World text.
Here the first parameter is the request which is coming to the web server and the second one is a stream to which a response will be passed.
Create Server
createServer method is used to create a web server. As a callback function, a request listener will be passed to it so we can send a response back and end the request.
one more thing, when an HTTP request sends to a particular port then the listener should watch the port and receives the request. So in this example, we’re listening to the port 1439 at 127.0.0.1 web address, a standard local web server address.
http.createServer(listener).listen(1439,'127.0.0.1');
Start Web Server
now execute the webserver.js using node command and a web server is ready to listen to the request and send the response back.
>$ node webserver.js
Test Web Server
Open your web browser and type http://localhost:1439 or http://127.0.0.1:1439/ in the web address and hit enter. You should see “Hello World” as a plain text response.
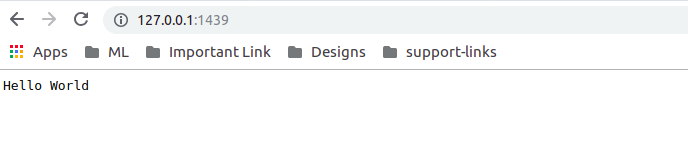
That’s it. You have created your own web server in node.js and you can send any type of response back e.g JSON, IMAGE or HTML.